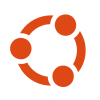
Data Platform Libs
- Canonical
- Databases
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/stable | 81 | 19 Nov 2024 | |
latest/edge | 82 | 04 Dec 2024 |
juju deploy data-platform-libs
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
charms.data_platform_libs.v0.s3
-
- Last updated 23 May 2024
- Revision Library version 0.5
A library for communicating with the S3 credentials providers and consumers.
This library provides the relevant interface code implementing the communication specification for fetching, retrieving, triggering, and responding to events related to the S3 provider charm and its consumers.
Provider charm
The provider is implemented in the s3-provider
charm which is meant to be deployed
alongside one or more consumer charms. The provider charm is serving the s3 credentials and
metadata needed to communicate and work with an S3 compatible backend.
Example:
from charms.data_platform_libs.v0.s3 import CredentialRequestedEvent, S3Provider
class ExampleProviderCharm(CharmBase):
def __init__(self, *args) -> None:
super().__init__(*args)
self.s3_provider = S3Provider(self, "s3-credentials")
self.framework.observe(self.s3_provider.on.credentials_requested,
self._on_credential_requested)
def _on_credential_requested(self, event: CredentialRequestedEvent):
if not self.unit.is_leader():
return
# get relation id
relation_id = event.relation.id
# get bucket name
bucket = event.bucket
# S3 configuration parameters
desired_configuration = {"access-key": "your-access-key", "secret-key":
"your-secret-key", "bucket": "your-bucket"}
# update the configuration
self.s3_provider.update_connection_info(relation_id, desired_configuration)
# or it is possible to set each field independently
self.s3_provider.set_secret_key(relation_id, "your-secret-key")
if __name__ == "__main__":
main(ExampleProviderCharm)
### Requirer charm
The requirer charm is the charm requiring the S3 credentials.
An example of requirer charm is the following:
Example:
```python
from charms.data_platform_libs.v0.s3 import (
CredentialsChangedEvent,
CredentialsGoneEvent,
S3Requirer
)
class ExampleRequirerCharm(CharmBase):
def __init__(self, *args):
super().__init__(*args)
bucket_name = "test-bucket"
# if bucket name is not provided the bucket name will be generated
# e.g., ('relation-{relation.id}')
self.s3_client = S3Requirer(self, "s3-credentials", bucket_name)
self.framework.observe(self.s3_client.on.credentials_changed, self._on_credential_changed)
self.framework.observe(self.s3_client.on.credentials_gone, self._on_credential_gone)
def _on_credential_changed(self, event: CredentialsChangedEvent):
# access single parameter credential
secret_key = event.secret_key
access_key = event.access_key
# or as alternative all credentials can be collected as a dictionary
credentials = self.s3_client.get_s3_credentials()
def _on_credential_gone(self, event: CredentialsGoneEvent):
# credentials are removed
pass
if __name__ == "__main__":
main(ExampleRequirerCharm)
Index
def
diff(
event: RelationChangedEvent,
bucket
)
Retrieves the diff of the data in the relation changed databag.
Arguments
relation changed event.
bucket of the databag (app or unit)
Returns
a Diff instance containing the added, deleted and changed keys from the event relation databag.
class BucketEvent
Description
Base class for bucket events. None
Methods
BucketEvent. bucket( self )
Description
Returns the bucket was requested. None
class CredentialRequestedEvent
Description
Event emitted when a set of credential is requested for use on this relation. None
class S3CredentialEvents
Description
Event descriptor for events raised by S3Provider. None
class S3Provider
Description
A provider handler for communicating S3 credentials to consumers. None
Methods
S3Provider. __init__( self , charm: CharmBase , relation_name: str )
S3Provider. fetch_relation_data( self )
Retrieves data from relation.
Returns
a dict of the values stored in the relation data bag for all relation instances (indexed by the relation id).
Description
This function can be used to retrieve data from a relation in the charm code when outside an event callback.
S3Provider. update_connection_info( self , relation_id: int , connection_data: dict )
Updates the credential data as set of key-value pairs in the relation.
Arguments
the identifier for a particular relation.
dict containing the key-value pairs that should be updated.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. relations( self )
Description
The list of Relation instances associated with this relation_name. None
S3Provider. set_bucket( self , relation_id: int , bucket: str )
Sets bucket name in application databag.
Arguments
the identifier for a particular relation.
the bucket name.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_access_key( self , relation_id: int , access_key: str )
Sets access-key value in application databag.
Arguments
the identifier for a particular relation.
the access-key value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_secret_key( self , relation_id: int , secret_key: str )
Sets the secret key value in application databag.
Arguments
the identifier for a particular relation.
the value of the secret key.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_path( self , relation_id: int , path: str )
Sets the path value in application databag.
Arguments
the identifier for a particular relation.
the path value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_endpoint( self , relation_id: int , endpoint: str )
Sets the endpoint address in application databag.
Arguments
the identifier for a particular relation.
the endpoint address.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_region( self , relation_id: int , region: str )
Sets the region location in application databag.
Arguments
the identifier for a particular relation.
the region address.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_s3_uri_style( self , relation_id: int , s3_uri_style: str )
Sets the S3 URI style in application databag.
Arguments
the identifier for a particular relation.
the s3 URI style.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_storage_class( self , relation_id: int , storage_class: str )
Sets the storage class in application databag.
Arguments
the identifier for a particular relation.
the storage class.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_tls_ca_chain( self , relation_id: int , tls_ca_chain )
Sets the tls_ca_chain value in application databag.
Arguments
the identifier for a particular relation.
the TLS Chain value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_s3_api_version( self , relation_id: int , s3_api_version: str )
Sets the S3 API version in application databag.
Arguments
the identifier for a particular relation.
the S3 version value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_delete_older_than_days( self , relation_id: int , days: int )
Sets the retention days for full backups in application databag.
Arguments
the identifier for a particular relation.
the value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Provider. set_attributes( self , relation_id: int , attributes )
Sets the connection attributes in application databag.
Arguments
the identifier for a particular relation.
the attributes value.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
class S3Event
Description
Base class for S3 storage events. None
Methods
S3Event. bucket( self )
Description
Returns the bucket name. None
S3Event. access_key( self )
Description
Returns the access key. None
S3Event. secret_key( self )
Description
Returns the secret key. None
S3Event. path( self )
Description
Returns the path where data can be stored. None
S3Event. endpoint( self )
Description
Returns the endpoint address. None
S3Event. region( self )
Description
Returns the region. None
S3Event. s3_uri_style( self )
Description
Returns the s3 uri style. None
S3Event. storage_class( self )
Description
Returns the storage class name. None
S3Event. tls_ca_chain( self )
Description
Returns the TLS CA chain. None
S3Event. s3_api_version( self )
Description
Returns the S3 API version. None
S3Event. delete_older_than_days( self )
Description
Returns the retention days for full backups. None
S3Event. attributes( self )
Description
Returns the attributes. None
class CredentialsChangedEvent
Description
Event emitted when S3 credential are changed on this relation. None
class CredentialsGoneEvent
Description
Event emitted when S3 credential are removed from this relation. None
class S3CredentialRequiresEvents
Description
Event descriptor for events raised by the S3Provider. None
class S3Requirer
Description
Requires-side of the s3 relation. None
Methods
S3Requirer. __init__( self , charm , relation_name: str , bucket_name )
Description
Manager of the s3 client relations. None
S3Requirer. fetch_relation_data( self )
Retrieves data from relation.
Returns
a dict of the values stored in the relation data bag for all relation instances (indexed by the relation id).
Description
This function can be used to retrieve data from a relation in the charm code when outside an event callback.
S3Requirer. update_connection_info( self , relation_id: int , connection_data: dict )
Updates the credential data as set of key-value pairs in the relation.
Arguments
the identifier for a particular relation.
dict containing the key-value pairs that should be updated.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
S3Requirer. get_s3_connection_info( self )
Description
Return the s3 credentials as a dictionary. None
S3Requirer. relations( self )
Description
The list of Relation instances associated with this relation_name. None