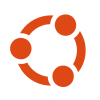
Data Platform Libs
- Canonical
- Databases
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/stable | 81 | 19 Nov 2024 | |
latest/edge | 82 | 04 Dec 2024 |
juju deploy data-platform-libs
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
charms.data_platform_libs.v0.database_provides
-
- Last updated 07 Jul 2023
- Revision Library version 0.4
[DEPRECATED] Relation provider side abstraction for database relation.
This library is a uniform interface to a selection of common database metadata, with added custom events that add convenience to database management, and methods to set the application related data.
It can be used as the main library in a database charm to handle relations with application charms or be extended/used as a template when creating a more complete library (like one that also handles the database and user creation using database specific APIs).
Following an example of using the DatabaseRequestedEvent, in the context of the database charm code:
from charms.data_platform_libs.v0.database_provides import DatabaseProvides
class SampleCharm(CharmBase):
def __init__(self, *args):
super().__init__(*args)
# Charm events defined in the database provides charm library.
self.provided_database = DatabaseProvides(self, relation_name="database")
self.framework.observe(self.provided_database.on.database_requested,
self._on_database_requested)
# Database generic helper
self.database = DatabaseHelper()
def _on_database_requested(self, event: DatabaseRequestedEvent) -> None:
# Handle the event triggered by a new database requested in the relation
# Retrieve the database name using the charm library.
db_name = event.database
# generate a new user credential
username = self.database.generate_user()
password = self.database.generate_password()
# set the credentials for the relation
self.provided_database.set_credentials(event.relation.id, username, password)
# set other variables for the relation event.set_tls("False")
As shown above, the library provides a custom event (database_requested) to handle the situation when an application charm requests a new database to be created. It's preferred to subscribe to this event instead of relation changed event to avoid creating a new database when other information other than a database name is exchanged in the relation databag.
Index
class DatabaseEvent
Description
Base class for database events. None
Methods
DatabaseEvent. database( self )
Description
Returns the database that was requested. None
DatabaseEvent. extra_user_roles( self )
Description
Returns the extra user roles that were requested. None
class DatabaseRequestedEvent
Description
Event emitted when a new database is requested for use on this relation. None
class DatabaseEvents
Database events.
Description
This class defines the events that the database can emit.
class DatabaseProvides
Description
Provides-side of the database relation. None
Methods
DatabaseProvides. __init__( self , charm: CharmBase , relation_name: str )
DatabaseProvides. fetch_relation_data( self )
Retrieves data from relation.
Returns
a dict of the values stored in the relation data bag for all relation instances (indexed by the relation id).
Description
This function can be used to retrieve data from a relation in the charm code when outside an event callback.
DatabaseProvides. relations( self )
Description
The list of Relation instances associated with this relation_name. None
DatabaseProvides. set_credentials( self , relation_id: int , username: str , password: str )
Set database primary connections.
Arguments
the identifier for a particular relation.
user that was created.
password of the created user.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
DatabaseProvides. set_endpoints( self , relation_id: int , connection_strings: str )
Set database primary connections.
Arguments
the identifier for a particular relation.
database hosts and ports comma separated list.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
DatabaseProvides. set_read_only_endpoints( self , relation_id: int , connection_strings: str )
Set database replicas connection strings.
Arguments
the identifier for a particular relation.
database hosts and ports comma separated list.
Description
This function writes in the application data bag, therefore, only the leader unit can call it.
DatabaseProvides. set_replset( self , relation_id: int , replset: str )
Set replica set name in the application relation databag.
Arguments
the identifier for a particular relation.
replica set name.
Description
MongoDB only.
DatabaseProvides. set_tls( self , relation_id: int , tls: str )
Set whether TLS is enabled.
Arguments
the identifier for a particular relation.
whether tls is enabled (True or False).
DatabaseProvides. set_tls_ca( self , relation_id: int , tls_ca: str )
Set the TLS CA in the application relation databag.
Arguments
the identifier for a particular relation.
TLS certification authority.
DatabaseProvides. set_uris( self , relation_id: int , uris: str )
Set the database connection URIs in the application relation databag.
Arguments
the identifier for a particular relation.
connection URIs.
Description
MongoDB, Redis, OpenSearch and Kafka only.
DatabaseProvides. set_version( self , relation_id: int , version: str )
Set the database version in the application relation databag.
Arguments
the identifier for a particular relation.
database version.