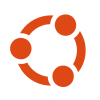
Data Platform Libs
- Canonical
- Databases
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/stable | 81 | 19 Nov 2024 | |
latest/edge | 82 | 04 Dec 2024 |
juju deploy data-platform-libs
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
charms.data_platform_libs.v0.database_requires
-
- Last updated 07 Jul 2023
- Revision Library version 0.6
[DEPRECATED] Relation 'requires' side abstraction for database relation.
This library is a uniform interface to a selection of common database metadata, with added custom events that add convenience to database management, and methods to consume the application related data.
Following an example of using the DatabaseCreatedEvent, in the context of the application charm code:
from charms.data_platform_libs.v0.database_requires import (
DatabaseCreatedEvent,
DatabaseRequires,
)
class ApplicationCharm(CharmBase):
# Application charm that connects to database charms.
def __init__(self, *args):
super().__init__(*args)
# Charm events defined in the database requires charm library.
self.database = DatabaseRequires(self, relation_name="database", database_name="database")
self.framework.observe(self.database.on.database_created, self._on_database_created)
def _on_database_created(self, event: DatabaseCreatedEvent) -> None:
# Handle the created database
# Create configuration file for app
config_file = self._render_app_config_file(
event.username,
event.password,
event.endpoints,
)
# Start application with rendered configuration
self._start_application(config_file)
# Set active status
self.unit.status = ActiveStatus("received database credentials")
As shown above, the library provides some custom events to handle specific situations, which are listed below:
— database_created: event emitted when the requested database is created. — endpoints_changed: event emitted when the read/write endpoints of the database have changed. — read_only_endpoints_changed: event emitted when the read-only endpoints of the database have changed. Event is not triggered if read/write endpoints changed too.
If it is needed to connect multiple database clusters to the same relation endpoint the application charm can implement the same code as if it would connect to only one database cluster (like the above code example).
To differentiate multiple clusters connected to the same relation endpoint the application charm can use the name of the remote application:
def _on_database_created(self, event: DatabaseCreatedEvent) -> None:
# Get the remote app name of the cluster that triggered this event
cluster = event.relation.app.name
It is also possible to provide an alias for each different database cluster/relation.
So, it is possible to differentiate the clusters in two ways.
The first is to use the remote application name, i.e., event.relation.app.name
, as above.
The second way is to use different event handlers to handle each cluster events. The implementation would be something like the following code:
from charms.data_platform_libs.v0.database_requires import (
DatabaseCreatedEvent,
DatabaseRequires,
)
class ApplicationCharm(CharmBase):
# Application charm that connects to database charms.
def __init__(self, *args):
super().__init__(*args)
# Define the cluster aliases and one handler for each cluster database created event.
self.database = DatabaseRequires(
self,
relation_name="database",
database_name="database",
relations_aliases = ["cluster1", "cluster2"],
)
self.framework.observe(
self.database.on.cluster1_database_created, self._on_cluster1_database_created
)
self.framework.observe(
self.database.on.cluster2_database_created, self._on_cluster2_database_created
)
def _on_cluster1_database_created(self, event: DatabaseCreatedEvent) -> None:
# Handle the created database on the cluster named cluster1
# Create configuration file for app
config_file = self._render_app_config_file(
event.username,
event.password,
event.endpoints,
)
...
def _on_cluster2_database_created(self, event: DatabaseCreatedEvent) -> None:
# Handle the created database on the cluster named cluster2
# Create configuration file for app
config_file = self._render_app_config_file(
event.username,
event.password,
event.endpoints,
)
...
Index
class DatabaseEvent
Description
Base class for database events. None
Methods
DatabaseEvent. endpoints( self )
Description
Returns a comma separated list of read/write endpoints. None
DatabaseEvent. password( self )
Description
Returns the password for the created user. None
DatabaseEvent. read_only_endpoints( self )
Description
Returns a comma separated list of read only endpoints. None
DatabaseEvent. replset( self )
Returns the replicaset name.
Description
MongoDB only.
DatabaseEvent. tls( self )
Description
Returns whether TLS is configured. None
DatabaseEvent. tls_ca( self )
Description
Returns TLS CA. None
DatabaseEvent. uris( self )
Returns the connection URIs.
Description
MongoDB, Redis, OpenSearch and Kafka only.
DatabaseEvent. username( self )
Description
Returns the created username. None
DatabaseEvent. version( self )
Returns the version of the database.
Description
Version as informed by the database daemon.
class DatabaseCreatedEvent
Description
Event emitted when a new database is created for use on this relation. None
class DatabaseEndpointsChangedEvent
Description
Event emitted when the read/write endpoints are changed. None
class DatabaseReadOnlyEndpointsChangedEvent
Description
Event emitted when the read only endpoints are changed. None
class DatabaseEvents
Database events.
Description
This class defines the events that the database can emit.
class DatabaseRequires
Description
Requires-side of the database relation. None
Methods
DatabaseRequires. __init__( self , charm , relation_name: str , database_name: str , extra_user_roles , relations_aliases )
Description
Manager of database client relations. None
DatabaseRequires. fetch_relation_data( self )
Retrieves data from relation.
Returns
a dict of the values stored in the relation data bag for all relation instances (indexed by the relation ID).
Description
This function can be used to retrieve data from a relation in the charm code when outside an event callback.
DatabaseRequires. relations( self )
Description
The list of Relation instances associated with this relation_name. None