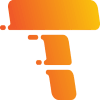
Tempo
- Canonical Observability
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/stable | 71 | 10 Sep 2024 | |
latest/stable | 9 | 16 Feb 2024 | |
latest/candidate | 75 | 10 Sep 2024 | |
latest/candidate | 9 | 12 Dec 2023 | |
latest/beta | 75 | 01 Aug 2024 | |
latest/beta | 9 | 12 Dec 2023 | |
latest/edge | 83 | 17 Sep 2024 | |
latest/edge | 9 | 31 Jul 2023 |
juju deploy tempo-k8s
Deploy Kubernetes operators easily with Juju, the Universal Operator Lifecycle Manager. Need a Kubernetes cluster? Install MicroK8s to create a full CNCF-certified Kubernetes system in under 60 seconds.
Platform:
charms.tempo_k8s.v1.charm_tracing
-
- Last updated 08 Oct 2024
- Revision Library version 1.17
This charm library has been transferred to the HA version of this charm.
The new owner is the tempo-coordinator-k8s
charm:
The new library (with its major version reset to 0) can be found at
https://charmhub.io/tempo-coordinator-k8s/libraries/charm_tracing
to install it:
charmcraft fetch-lib charms.tempo_coordinator_k8s.v0.charm_tracing
The API is unchanged, so you can search and replace the path to swap the old lib with the new one.
Index