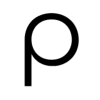
Parca
- Canonical Observability
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/stable | 128 | 14 Sep 2023 | |
latest/beta | 137 | 29 Feb 2024 | |
latest/edge | 154 | 29 Jul 2024 |
juju deploy parca
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
22.04
20.04
charms.parca.v0.parca_scrape
-
- Last updated 13 Jan 2025
- Revision Library version 0.6
The parca
machine charm has been deprecated.
This library has been transferred to the parca-k8s
charm.
If you are using this library, please replace it with the new one:
charmcraft fetch-lib charms.parca_k8s.v0.parca_scrape
Index