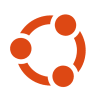
MongoDB
- Canonical
- Databases
Channel | Revision | Published | Runs on |
---|---|---|---|
6/stable | 199 | 04 Oct 2024 | |
6/candidate | 199 | 04 Oct 2024 | |
6/beta | 199 | 04 Oct 2024 | |
6/edge | 211 | 10 Mar 2025 | |
5/stable | 117 | 20 Apr 2023 | |
5/candidate | 117 | 20 Apr 2023 | |
5/edge | 139 | 21 Nov 2023 | |
5/edge | 109 | 06 Mar 2023 | |
3.6/stable | 100 | 28 Apr 2023 | |
3.6/candidate | 100 | 13 Apr 2023 | |
3.6/edge | 100 | 03 Feb 2023 |
juju deploy mongodb --channel 6/stable
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
charms.mongodb.v1.mongos
-
- Last updated 21 Aug 2024
- Revision Library version 1.7
Code for interactions with MongoDB.
Index
class NotEnoughSpaceError
Description
Raised when there isn't enough space to movePrimary. None
class ShardNotInClusterError
Description
Raised when shard is not present in cluster, but it is expected to be. None
class ShardNotPlannedForRemovalError
Description
Raised when it is expected that a shard is planned for removal, but it is not. None
class NotDrainedError
Description
Raised when a shard is still being drained. None
class BalancerNotEnabledError
Description
Raised when balancer process is not enabled. None
class MongosConnection
In this class we create connection object to Mongos.
Description
Real connection is created on the first call to Mongos. Delayed connectivity allows to firstly check database readiness and reuse the same connection for an actual query later in the code.
Connection is automatically closed when object destroyed. Automatic close allows to have more clean code.
Note that connection when used may lead to the following pymongo errors: ConfigurationError, ConfigurationError, OperationFailure. It is suggested that the following pattern be adopted when using MongoDBConnection:
with MongoMongos(self._mongos_config) as mongo: try: mongo.<some operation from this class> except ConfigurationError, OperationFailure: <error handling as needed>
Methods
MongosConnection. __init__( self , config: MongoConfiguration , uri , direct )
A MongoDB client interface.
Arguments
MongoDB Configuration object.
allow using custom MongoDB URI, needed for replSet init.
force a direct connection to a specific host, avoiding reading replica set configuration and reconnection.
MongosConnection. get_shard_members( self )
Gets shard members.
Description
Returns: A set of the shard members as reported by mongos.
Raises: ConfigurationError, OperationFailure
MongosConnection. add_shard( self , shard_name , shard_hosts , shard_port )
Adds shard to the cluster.
Description
Raises: ConfigurationError, OperationFailure
MongosConnection. pre_remove_checks( self , shard_name )
Performs a series of checks for removing a shard from the cluster.
Description
Raises ConfigurationError, OperationFailure, NotReadyError, ShardNotInClusterError, BalencerNotEnabledError
MongosConnection. start_and_wait_for_balancer( self )
Turns on the balancer and waits for it to be running.
Description
Starting the balancer doesn't guarantee that is is running, wait until it starts up.
Raises: BalancerNotEnabledError
MongosConnection. remove_shard( self , shard_name: str )
Removes shard from the cluster.
Description
Raises: ConfigurationError, OperationFailure, NotReadyError, NotEnoughSpaceError, ShardNotInClusterError, BalencerNotEnabledError
MongosConnection. get_databases_for_shard( self , primary_shard )
Returns a list of databases using the given shard as a primary shard.
Description
In Sharded MongoDB clusters, mongos selects the primary shard when creating a new database by picking the shard in the cluster that has the least amount of data. This means that:
- There can be multiple primary shards in a cluster.
- Until there is data written to the cluster there is effectively no primary shard.
MongosConnection. is_any_draining( self , ignore_shard: str )
Returns true if any shard members is draining.
Arguments
current state of shard cluster status as reported by mongos.
shard to ignore
Description
Checks if any members in sharded cluster are draining data.
MongosConnection. is_ready( self )
Is mongos ready for services requests.
Description
Returns: True if services is ready False otherwise. Retries over a period of 60 seconds times to allow server time to start up.
Raises: ConfigurationError, ConfigurationError, OperationFailure
MongosConnection. are_all_shards_aware( self )
Description
Returns True if all shards are shard aware. None
MongosConnection. is_shard_aware( self , shard_name: str )
Description
Returns True if provided shard is shard aware. None
MongosConnection. get_db_size( self , database_name , primary_shard )
Description
Returns the size of a DB on a given shard in bytes. None
MongosConnection. get_shard_with_most_available_space( self , shard_to_ignore )
Returns the shard in the cluster with the most available space and the space in bytes.
Description
Algorithm used was similar to that used in mongo in selectShardForNewDatabase
:
https://github.com/mongodb/mongo/blob/6/0/src/mongo/db/s/config/sharding_catalog_manager_database_operations.cpp#L68-L91
MongosConnection. get_draining_shards( self )
Description
Returns a list of the shards currently draining. None