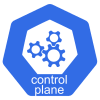
Kubernetes
- Canonical Kubernetes
Channel | Revision | Published | Runs on |
---|---|---|---|
latest/edge | 713 | Today | |
latest/edge | 712 | Today | |
latest/edge | 711 | Today | |
latest/edge | 710 | Today | |
latest/edge | 578 | 19 Mar 2025 | |
latest/edge | 576 | 19 Mar 2025 | |
1.32/stable | 456 | 25 Mar 2025 | |
1.32/stable | 459 | 25 Mar 2025 | |
1.32/stable | 458 | 25 Mar 2025 | |
1.32/stable | 461 | 25 Mar 2025 | |
1.32/stable | 460 | 25 Mar 2025 | |
1.32/stable | 457 | 25 Mar 2025 | |
1.32/candidate | 456 | 20 Feb 2025 | |
1.32/candidate | 459 | 20 Feb 2025 | |
1.32/candidate | 457 | 20 Feb 2025 | |
1.32/candidate | 460 | 20 Feb 2025 | |
1.32/candidate | 458 | 20 Feb 2025 | |
1.32/candidate | 461 | 20 Feb 2025 | |
1.32/beta | 709 | Today | |
1.32/beta | 708 | Today | |
1.32/beta | 707 | Today | |
1.32/beta | 706 | Today | |
1.32/beta | 705 | Today | |
1.32/beta | 704 | Today | |
1.31/candidate | 142 | 11 Dec 2024 | |
1.31/candidate | 141 | 27 Nov 2024 | |
1.30/beta | 65 | 23 May 2024 |
juju deploy k8s --channel 1.32/stable
Deploy universal operators easily with Juju, the Universal Operator Lifecycle Manager.
Platform:
charms.k8s.v0.k8sd_api_manager
-
- Last updated 01 Mar 2024
- Revision Library version 0.2
Module for managing k8sd API interactions.
This module provides a high-level interface for interacting with K8sd. It simplifies tasks such as token management and component updates.
The core of the module is the K8sdAPIManager, which handles the creation and management of HTTP connections to interact with the k8sd API. This class utilises different connection factories (UnixSocketConnectionFactory and HTTPConnectionFactory) to establish connections through either Unix sockets or HTTP protocols.
Example usage for creating a join token for K8sd:
try:
factory = UnixSocketConnectionFactory('/path/to/socket')
api_manager = K8sdAPIManager(factory)
join_token = api_manager.create_join_token('node-name')
except K8sdAPIManagerError as e:
logger.error("An error occurred: %s", e.message)
Similarly, the module allows for requesting authentication tokens and managing K8s components.
Index
class K8sdAPIManagerError
Description
Base exception for K8sd API Manager errors. None
class K8sdConnectionError
Description
Raised when there is a connection error. None
class InvalidResponseError
Description
Raised when the response is invalid or unexpected. None
class BaseRequestModel
Base model for k8s request responses.
Attributes
Methods
BaseRequestModel. check_status_code( cls , v )
Validate the status_code field.
Arguments
The value of the status_code field to validate.
Returns
The validated status code if it is 200.
BaseRequestModel. check_error_code( cls , v , values )
Validate the error_code field.
Arguments
The value of the error_code field to validate.
The values dictionary.
Returns
The validated error code if it is 0.
class EmptyResponse
Description
Response model for request that do not expect any return value. None
class TokenMetadata
Model representing metadata for a token.
Attributes
class AuthTokenResponse
Response model for Kubernetes authentication token requests.
Attributes
class CreateJoinTokenResponse
Response model for join token creation requests.
Attributes
class ClusterMember
Represents a member in the k8sd cluster.
Attributes
class ClusterComponent
Represents a component in the k8sd cluster.
Attributes
class ClusterStatus
Represents the overall status of the k8sd cluster.
Attributes
class ClusterMetadata
Metadata containing status information about the k8sd cluster.
Attributes
class GetClusterStatusResponse
Response model for getting the status of the k8sd cluster.
Attributes
class UnixSocketHTTPConnection
Description
HTTP connection over a Unix socket. None
Methods
UnixSocketHTTPConnection. __init__( self , unix_socket: str , timeout: int )
Initialise the UnixSocketHTTPConnection.
Arguments
Path to the Unix socket.
Connection timeout in seconds.
UnixSocketHTTPConnection. connect( self )
Establish a connection to the server using a Unix socket.
class ConnectionFactory
Description
Abstract factory for creating connection objects. None
Methods
ConnectionFactory. create_connection( self )
Create a new connection instance.
class UnixSocketConnectionFactory
Description
Concrete factory for creating Unix socket connections. None
Methods
UnixSocketConnectionFactory. __init__( self , unix_socket: str , timeout: int )
Initialize a new instance of UnixSocketConnectionFactory.
Arguments
The file path to the Unix socket.
The timeout for the connection in seconds. Defaults to 30 seconds.
UnixSocketConnectionFactory. create_connection( self )
Create and manage a Unix socket HTTP connection.
Returns
The created Unix socket HTTP connection.
class HTTPConnectionFactory
Description
Concrete factory for creating HTTP connections. None
Methods
HTTPConnectionFactory. __init__( self , host: str , port , timeout: int )
Initialize a new instance of HTTPConnectionFactory.
Arguments
Hostname for the HTTP connection.
Port for the HTTP connection.
The timeout for the connection in seconds. Defaults to 30 seconds.
HTTPConnectionFactory. create_connection( self )
Create and manage an HTTP connection.
Returns
The created HTTP connection.
class K8sdAPIManager
Description
Manager for K8sd API interactions. None
Methods
K8sdAPIManager. __init__( self , factory: ConnectionFactory )
Initialise the K8sdAPIManager.
Arguments
An instance of a connection factory that will be used to create connections. This factory determines the type of connection (e.g., Unix socket or HTTP).
K8sdAPIManager. create_join_token( self , name: str , worker: bool )
Create a join token.
Arguments
Name of the node.
Whether the node should join as control-plane or worker.
Returns
The generated join token if successful.
K8sdAPIManager. join_cluster( self , name: str , address: str , token: str )
Join a node to the k8s cluster.
Arguments
Name of the node.
address to which k8sd should be bound
The join token for this node.
K8sdAPIManager. remove_node( self , name: str , force: bool )
Remove a node from the cluster.
Arguments
Name of the node that should be removed.
Forcibly remove the node
K8sdAPIManager. enable_component( self , name: str , enable: bool )
Enable or disable a k8s component.
Arguments
Name of the component.
True to enable, False to disable the component.
K8sdAPIManager. is_cluster_bootstrapped( self )
Check if K8sd has been bootstrapped.
Returns
True if the cluster has been bootstrapped, False otherwise.
K8sdAPIManager. is_cluster_ready( self )
Check if the Kubernetes cluster is ready.
Returns
True if the cluster is ready, False otherwise.
Description
The cluster is ready if at least one k8s node is in READY state.
K8sdAPIManager. check_k8sd_ready( self )
Description
Check if k8sd is ready. None
K8sdAPIManager. bootstrap_k8s_snap( self , name: str , address: str )
Bootstrap the k8s cluster.
Arguments
name of the node
address to which k8sd should be bound
K8sdAPIManager. request_auth_token( self , username: str , groups )
Request a Kubernetes authentication token.
Arguments
Username for which the token is requested.
Groups associated with the user.
Returns
The authentication token.